Exception Handling
In this section, we study Exception Handling.
Handling Exceptions
Throwing Exceptions
Advanced Topics: Binary Files, Random Access Files,
and Object Serialization (Optional)
Handling Exceptions
An exception is a run-time error that can occur during the normal course of program execution.When an exception occurs, we say an exception is thrown.
Unhandled exceptions will crash the program.
Program Reliability:
We expect the program to produce correct result for valid input.
Program Robustness:
We expect the program to run under various conditions such as:
- Invalid input, 5/0, array index out of bound, corrupted data file, and etc.
Exception handling is to improve the robustness.
We should write robust and fault tolerant programs that can handle exceptions.
When an exception occurs, the normal sequence of program flow is terminated immediately and the exception handling code should be executed.
When the matching exception handling code is executed, we say the thrown exception is caught.
Java has a well designed exception handling mechanism.
Exception handling in Java enables us to remove exception handling code from the “main line” of the program’s execution.
It improves program clarity.
Exception Classes
An exception is an object.
Exception objects are created from classes in the Java API hierarchy of exception classes as shown in the following figure:
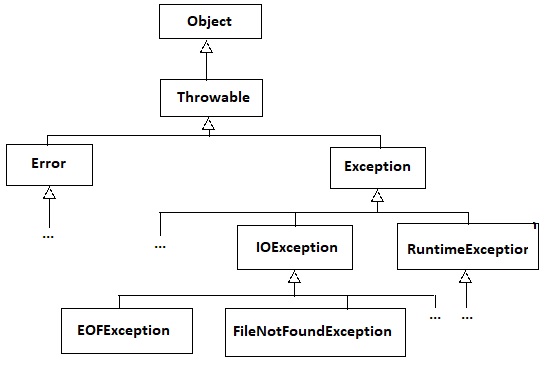
All of the exception classes in the hierarchy are derived from the Throwable class.
Error and Exception are derived from the Throwable class.
Classes that are derived from Error are for exceptions that are thrown when critical errors occur, such as an internal error in the Java Virtual Machine, or running out of memory.
Applications should not try to handle these errors because they are the result of a serious condition.
We learn how to handle the exceptions that are instances of classes that are derived from the Exception class.
Refer to Java API Documentation for more details.
Handling Exceptions
Java allows us to create exception handlers.
An exception handler is a section of code that can handle the exception.
The default exception handler prints an error message and crashes the program.
Java Exception Handling Mechanism
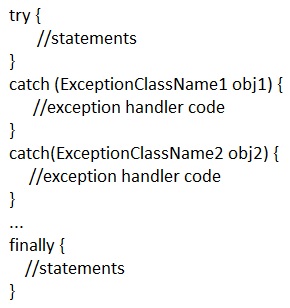
The try { } block contains statements that might cause exceptions and statements that should not execute if an exception occurs.
At least one catch() block (exception handler / routine) or a finally{} block must immediately follow the try {} block.
Each catch() has an exception parameter that identifies the exception type.
catch (ExceptionType ParameterName)
ExceptionType is the name of an exception class and ParameterName is a variable name which will reference the exception object if the code in the try block throws an exception.
finally{} block is optional. Normally, it is for code that releases resources to prevent “resource leaks”.
If no catch() block, the finally{ } block is required.
If an exception occurs in a try {} block, the try {} block terminates immediately (throw point) and program control transfers to the first catch().
The program locates the matching catch() by comparing the thrown exception’s type with each catch() block’s parameter type.
When a match occurs, the code contained within the catch() executes.
If no exceptions occur in the try {} block, the program ignores all catch() blocks.
The finally{ } executes regardless whether an exception occurs or not.
Order of catch Blocks / Polymorphic References To Exceptions
When handling exceptions, you can use a polymorphic reference as a parameter in the catch clause.
Most exceptions are derived from the Exception class.
A catch clause that uses a parameter variable of the Exception type is capable of catching any exception that is derived from the Exception class.
//This section of code will cause compiling error.
catch (Exception e) {
//statements
}
catch (ArithmeticException e) {
//statements
}
//The first catch block will catch any types of exceptions.
//The second catch block will never be reached, so it causes compiling error.
The catch clauses must be listed from the most specific to the most general.catch (Exception e) {
//statements
}
catch (ArithmeticException e) {
//statements
}
//The first catch block will catch any types of exceptions.
//The second catch block will never be reached, so it causes compiling error.
A try statement may have only one catch clause for each specific type of exception.
Checked and Unchecked Exceptions
Two types of exceptions: checked and unchecked.
Unchecked exceptions: Class RuntimeException and all its subclasses.
Checked exceptions: All other Exceptions except RuntimeException.
The Java compiler enforces exception handling for all checked exceptions.
The Java compiler does not require exception handling for all unchecked exceptions, because unchecked exceptions can be prevented by proper coding. For example: divided by zero, array out of bound, and string out of bound.
The code section shown below does not handle the exception, just prevent the compiling errors.
public static void main(String[] args) throws FileNotFoundException {
Scanner inFile = new Scanner(new FileReader("test.txt"));
}
Scanner inFile = new Scanner(new FileReader("test.txt"));
}
The code section below is designed to handle the FileNotFoundException if it is thrown.
try {
Scanner inFile = new Scanner(new FileReader("test.txt"));
}
catch (FileNotFoundException e){
System.out.println("File not found.");
}
Scanner inFile = new Scanner(new FileReader("test.txt"));
}
catch (FileNotFoundException e){
System.out.println("File not found.");
}
Retrieving the Default Error Message
Each exception object has a method named getMessage that can be used to retrieve the default error message for the exception.
catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
System.out.println(e.getMessage());
}
Throwing Exceptions
You can write code that throws one of the standard Java exceptions, or an instance of a custom exception class that you have designed.The throw statement is used to manually throw an exception.
throw new Exception("Negative Number");
The throw statement causes an exception object to be created and thrown.
The argument contains a custom error message that can be retrieved from the exception object’s getMessage() method.
If you do not pass a message to the constructor, the exception will have a null message.
Note: Do not confuse the throw statement with the throws clause.
The Stack Trace
The call stack is an internal list of all the methods that are currently executing.
A stack trace is a list of all the methods in the call stack.
It indicates the method that was executing when an exception occurred and all of the methods that were called in order to execute that method.
Creating Customized Exception Classes
You can create your own exception classes by deriving them from the Exception class or one of its derived classes.
Using the @exception Tag in Documentation Comments
@exception ExceptionName Description