Class Roster Program
Description
This program uses the OOP inheritance concept to design classes to represent different types of student.Suppose a college course enrolled with both undergraduate and graduate students.
Each student, either undergraduate or graduate, has the name, three test scores, and the final course grade as data members.
The final course grade, either pass or fail, is determined differently.
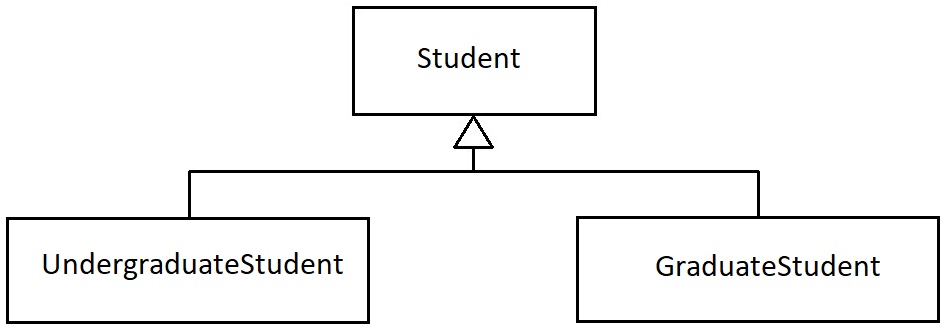
The superclass Student defines the common features that apply to any types of student.
Data members: name, tests, grade
constructors
getters and setters
determineGrade method
toString method
constructors
getters and setters
determineGrade method
toString method
The subclasses UndergraduateStudent and GraduateStudent inherit the superclass Student's features and override the determineGrade() method.
Undergraduate: average test score >= 70
Graduate: average test score >=80
Graduate: average test score >=80
This problem was originally presented as an example in An Introduction to Object-Oriented Programming with Java book. Authors: C. Thomas Wu
The requirements are revised to meet our purpose.
Associated Concepts:
OOP - Inheritance
Algorithm:
Class diagrams:
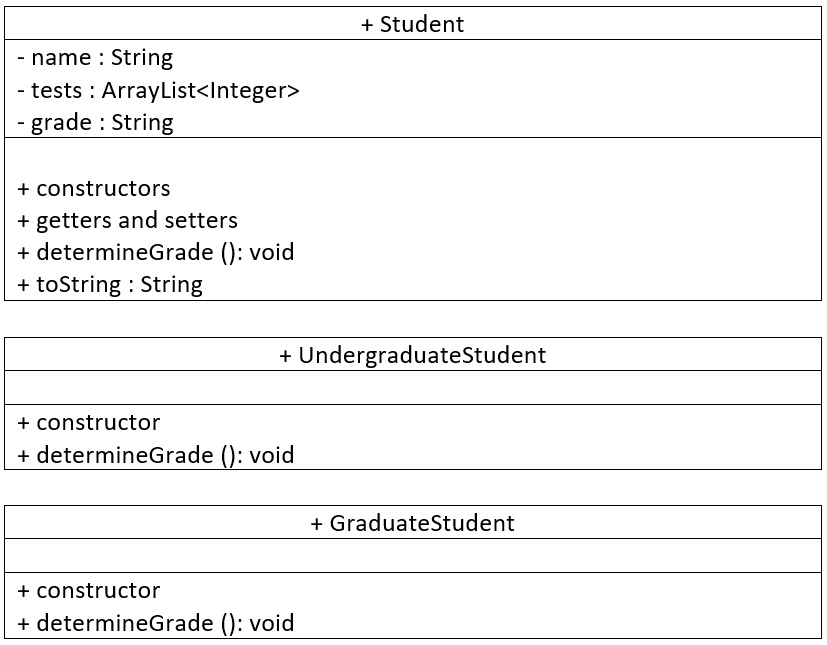
Client program:
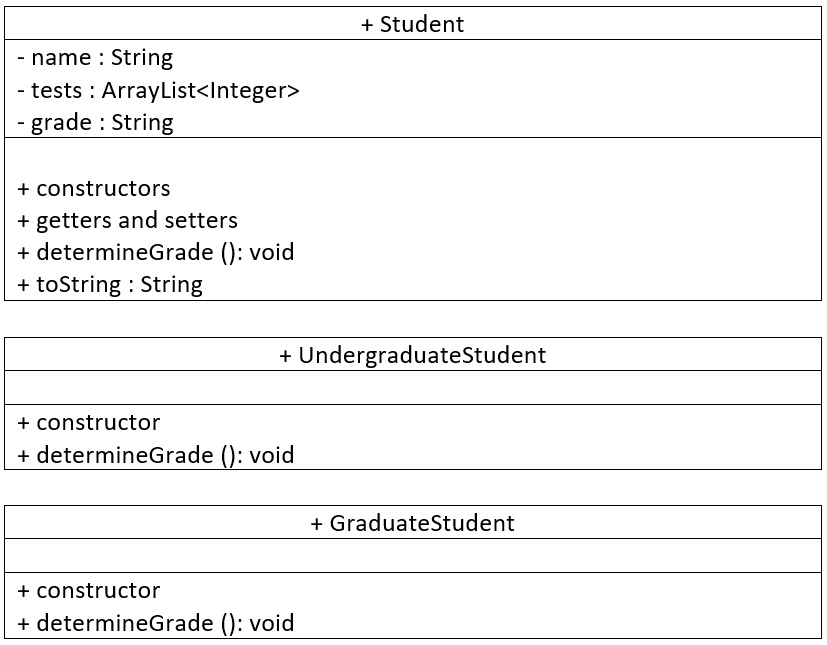
Client program:
The client program creates objects of different types of
students and add three test scores to each object.
It then uses the object references to call determineGrade() method and prints the objects.
It then uses the object references to call determineGrade() method and prints the objects.
Source Code
Superclass Student class
Subclass GraduateStudent class

subclass UndergraduateStudent class

Client program

Sample Run
