Java Fundamentals
In Chapter 1 and 2, we study the following Java fundamental concepts:
Class
Method
Output Statement
Escape Sequence
Comment
Variables
Data Types
Constants
Arithmetic Operators
Data Conversion
Standard Input
String Class
JOptionPane Class
Math Class
Scope
Style, Format, and Documentation
Class
The base of a Java program is a class.It is surrounded by a pair of curly braces { } to indicate the beginning and ending of the program.
public class FirstProgram {
A Java source code file can have more than one class. But only one
of them may be public.
Java Code
} In COMP2243, we write only one public class in the source code and the file name of the source code must match the public class name.
For example: The class named HelloWorld must be in a file named HelloWorld.java.
Method
The code or Java statements are written within individual methods.A method has a sequence of code to perform a task.
A large program can have many methods.
In the beginning of the course, we write the program with only one method, the main() method, that is the entry point of the program.
Basically the program execution starts at the main() method.
Later in Methods section, we will learn how to incorporate multiple methods into the program.
public class FirstProgram {
public static void main(String[] args) {
Java Code
}
}
public static void main(String[] args) {
Java Code
}
}
Output Statement
System.out.println( ) statement prints the contents in the parentheses on the computer's monitor (standard output device), followed by a newline.System.out.print( ) statement prints the contents in the parentheses on the computer's monitor (standard output device), without a following newline.
System.out.println("Welcome");
System.out.print("Java Programming");
System.out.print("Java Programming");
Comment
Multi-line comments are written between /* and */.A single line comment is written after //.
/* Multi-line comments:
Comments are not
executable statements.
*/
// This is a single line comment.
Comments are not
executable statements.
*/
// This is a single line comment.
Escape Sequence
Escape sequences are special characters. With them, programs can print characters that otherwise would be unprintable.
\n newline
\t tab
\\ backslash
\” double quote
\t tab
\\ backslash
\” double quote
System.out.println("Welcome to RCTC\n");
System.out.println("My name is \"John\"");
System.out.println("My name is \"John\"");
Variables and Literals
A variable is a named space in the computer’s main memory.An actual data value can be assigned to a variable which can be altered during the program execution.
A literal is the actual data value.
Primitive Data Type
There are two categories of data types: primitive and reference.Let's study the primitive data type first. Reference type will be studied in String Class section.
Java has 8 primitives:
Data Type | Size | Purpose |
---|---|---|
byte | one-byte | integer |
short | two-byte | integer |
int | four-byte | integer |
long | eight-byte | integer |
float | four-byte | floating-point value |
double | eight-byte | floating-point value |
char | two-byte | single character in Unicode |
boolean | Boolean value: true / false |
int quantity, count;
double price, tax;
char status;
boolean flag;
The boolean data type can have two possible values: true or false.double price, tax;
char status;
boolean flag;
The char data type can only store a single character that is enclosed in single quote marks.
Unicode vs. ASCII code
ASCII code – 8 bits, 256 symbols in total
Unicode - 16 bits, 65536 possible symbols
- The Unicode standard currently contains 34,168 distinct characters.
- ‘\unn’ is the Unicode format encoding (nn: the hexadecimal number representing the character)
Variable Assignment
The variables must be declared before they can be used.
The assigned value must be compatible with the variable’s declared type.
int quantity = 25, count;
double price = 12.99, discount;
char status;
boolean flag;
Variable Initializationdouble price = 12.99, discount;
char status;
boolean flag;
The variables must be initialized prior to be used, otherwise compiling error will occur.
count = 5;
discount = 2.5;
status = 'F';
flag = true;
Naming Rules and Conventiondiscount = 2.5;
status = 'F';
flag = true;
Variable names can only contains letters, digits, _, and $.
However, they cannot start with a digit.
int _numbers;
double $price;
int 5item; //illegal
Variable names should be meaningful and descriptive.double $price;
int 5item; //illegal
Naming Conventions
Variable names should begin with a lower case letter and then switch to upper case at each word change.
double taxRate;
Class names should start with an upper case letter.
public class HelloWorld
Constants
A constant remains the same value throughout the program execution.Once initialized with a value, constants cannot be changed.
We can use named constants rather than hard-coded values throughout the program.
By convention, constants use all upper case letters and words are separated by the underscore.
final double DISCOUNT_RATE = 0.25;
Arithmetic Operators
+ addition
- subtraction
* multiplication
/ division
% modulus
Dividing two integers using the / operator produces the quotient as the result.- subtraction
* multiplication
/ division
% modulus
Dividing two integers using the % operator produces the remainder as the result.
5 / 2 = 2
5 % 2 = 1
Operator Precedence5 % 2 = 1
((( 3 + 4 ) * 3 ) + (( 5*2) / 4)) + 6
Combined Assignment Operators
+=, -=, *=, /=, %=
x += 5; //Equivalent to x = x + 5;
Increment and Decrement Operators
++ Increment by 1
-- Decrement by 1
-- Decrement by 1
int n = 5;
n++; //Equivalent to n = n + 1
n--; //Equivalent to n = n - 1
Prefix ( ++n) vs. postfix ( n++ )n++; //Equivalent to n = n + 1
n--; //Equivalent to n = n - 1
When they are used independently, the effect is the same.
The difference is when they are used in an expression.
int x, y;
x = 5;
y = ++x; //increment x first, then use the new value of x to assign to y
System.out.println(x); //6 is printed.
System.out.println(y); //6 is printed.
x = 5;
y = ++x; //increment x first, then use the new value of x to assign to y
System.out.println(x); //6 is printed.
System.out.println(y); //6 is printed.
int x, y;
x = 5;
y = x++; //assign the current value of x to y first, then increment x by 1
System.out.println(x); //6 is printed.
System.out.println(y); //5 is printed.
x = 5;
y = x++; //assign the current value of x to y first, then increment x by 1
System.out.println(x); //6 is printed.
System.out.println(y); //5 is printed.
Data Conversion
Conversion from a lower rank data type to a high rank data type is automatic. It is call implicit conversion.
int n = 5;
double d;
d = n; //Implicit conversion
System.out.println(d); //5.0 is printed.
Conversion from a higher rank data type to a lower rank data type
requires casting. It is called explicit conversion.double d;
d = n; //Implicit conversion
System.out.println(d); //5.0 is printed.
int n;
double d = 2.56;
n = (int)d; //Explicit conversion
System.out.println(n); //2 is printed and the decimal part value is lost.
Mixed Operationsdouble d = 2.56;
n = (int)d; //Explicit conversion
System.out.println(n); //2 is printed and the decimal part value is lost.
int n = 5;
double d = 2.56;
double x = d + n; //n is converted to 5.0 implicitly.
System.out.println(x); //7.56 is printed.
double d = 2.56;
double x = d + n; //n is converted to 5.0 implicitly.
System.out.println(x); //7.56 is printed.
String Class
String class is in Java Standard Library.Java Standard Library has a large number of classes. Refer to Java API Documentation.
A string is a sequence of characters surrounded by a pair double quotes.
String s1 = " ";
String school = "RCTC";
String course = "COMP 2243";
Strings are reference type. String school = "RCTC";
String course = "COMP 2243";
A String variable is a reference which refers to a String object in main memory.
String greeting = "Hello";
The variable greeting refers to the String object "Hello"
which has a starting memory address. The variable itself contains the value of this memory address.
If a new value "Hi" is assigned to greeting, "Hi" object is created in another memory location and its starting address is assigned to greeting.
String greeting = "Hi";
What happened to "Hello" object? Answer: It becomes a garbage.
Java has an automatic garbage collector which is a background program to collect garbage.
Any object without a reference becomes a garbage.
String Concatenate Operator
+ is the concatenate operator when used to join any data item to a string.
If one of the operand is a string, + operator will join two operands together to form a new string.
System.out.println("COMP " + 2243);
System.out.println("Answer: " + 5);
System.out.println("Answer: " + ( n1 + n2) );
String OrganizationSystem.out.println("Answer: " + 5);
System.out.println("Answer: " + ( n1 + n2) );
A string is stored in an array which is a data structure to store multiple data items.
We will study the concept of array later in detail. For now, let's study the basics of array.
An array is a container with many cells, each is capable of storing one data item.
Each cell has an index, with 0 as the first cell, 1 as the second cell, and etc.
For example:
String name;
name = "John Doe";
name = "John Doe";
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
'J' | 'o' | 'h' | 'n' | ' ' | 'D' | 'o' | 'e' |
String Methods
String class has many methods to manipulate strings. Refer to Java API Documentation.
Let's study a few of methods in String class.
String name = "John Doe";
int x = name.length(); //It returns the size of string: 8.
char c = name.charAt(2); //It returns the character at index 2: h.
int k = name.indexOf("Doe"); //It returns the index of "Doe": 5.
String firstName = name.substring(0, 4); //It returned a substring from 0 and up to but not including 4: John.
String lastName = name.substring(5); //It returns a substring from 5 to the end: Doe.
String nameCap = name.toUpperCase(); //It returns the name in uppercase: JOHN DOE.
int x = name.length(); //It returns the size of string: 8.
char c = name.charAt(2); //It returns the character at index 2: h.
int k = name.indexOf("Doe"); //It returns the index of "Doe": 5.
String firstName = name.substring(0, 4); //It returned a substring from 0 and up to but not including 4: John.
String lastName = name.substring(5); //It returns a substring from 5 to the end: Doe.
String nameCap = name.toUpperCase(); //It returns the name in uppercase: JOHN DOE.
Standard Input
We use the Scanner object to input data from the computer's keyboard (standard input device).
import java.util.Scanner;
public class FirstProgram {
public static void main(String[] args) {
Scanner keyboard = new Scanner (System.in); //create a Scanner object called keyboard
int num;
double price;
String title, name;
char c;
num = keyboard.nextInt(); //read an integer and store it in variable num
price = keyboard.nextDouble(); //read a decimal value and store it in variable price
title = keyboard.next(); //read a string excluding blank spaces and store it in variable title
name = keyboard.nextLine(); //read a string including blank spaces and store it in variable name
c = keyboard.next().charAt(0); //read a string, get the first letter, and store it in variable c
}
Calling nextLine() with calls to other methodspublic class FirstProgram {
public static void main(String[] args) {
Scanner keyboard = new Scanner (System.in); //create a Scanner object called keyboard
int num;
double price;
String title, name;
char c;
num = keyboard.nextInt(); //read an integer and store it in variable num
price = keyboard.nextDouble(); //read a decimal value and store it in variable price
title = keyboard.next(); //read a string excluding blank spaces and store it in variable title
name = keyboard.nextLine(); //read a string including blank spaces and store it in variable name
c = keyboard.next().charAt(0); //read a string, get the first letter, and store it in variable c
}
System.out.print("Enter a decimal value: ");
double value = keyboard.nextDouble();
System.out.print("Enter a string: ");
name = keyboard.nextLine();
For the nextDouble() method, the user enters a decimal value and presses
the enter key. The enter key (newline) is stored in the buffer.double value = keyboard.nextDouble();
System.out.print("Enter a string: ");
name = keyboard.nextLine();
For the nextLine() method, it doesn't skip the newline in the buffer. Instead, it accepts it and terminates right away because the nextLine() method is designed to stop at the newline. So the user doesn't have a chance to enter anything.
To fix the problem, add another nextLine() method to remove the newline character in the buffer.
System.out.print("Enter a decimal value: ");
double value = keyboard.nextDouble();
keyboard.nextLine(); //remove the newline in the buffer
System.out.print("Enter a string: ");
name = keyboard.nextLine();
double value = keyboard.nextDouble();
keyboard.nextLine(); //remove the newline in the buffer
System.out.print("Enter a string: ");
name = keyboard.nextLine();
JOptionPane Class
There are two graphical dialog boxes in JOptionPane class.
Input dialog box
Message dialog box
Message dialog box
Input Dialog: a dialog box that prompts the user for input.
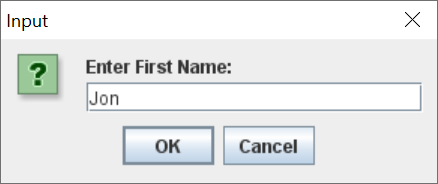
It has a text field, an Ok button and a Cancel button.
If the Ok button is pressed, the dialog returns the user’s input.
If the Cancel button is pressed, the dialog returns null.
The input dialog returns a string. We can parse it to a numerical value.
If the Ok button is pressed, the dialog returns the user’s input.
If the Cancel button is pressed, the dialog returns null.
The input dialog returns a string. We can parse it to a numerical value.
String name = JOptionPane.showInputDialog("Enter product name: ");
double price = Double.parseDouble(JOptionPane.showInputDialog( "Enter product price: "));
int number = Integer.parseInt(JOptionPane.showInputDialog( "Enter product quantity: "));
double price = Double.parseDouble(JOptionPane.showInputDialog( "Enter product price: "));
int number = Integer.parseInt(JOptionPane.showInputDialog( "Enter product quantity: "));
Message Dialog: a dialog box that displays a message.
JOptionPane.showMessageDialog(null, name + ": " + price);
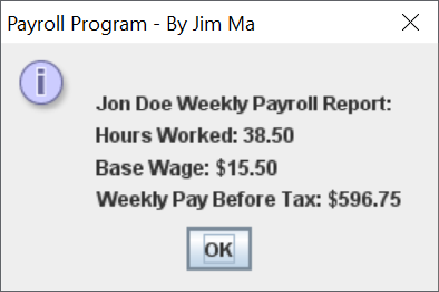
Programs with JOptionPane do not exit immediately even though the main() method is ended.
We can use System.exit(0) to exit the program.
System.exit(0);
This argument is the exit code used by the operating system.
0 - Success
1 - Error
1 - Error
Math Class
Math class is a class in Java Standard Library. It has many methods, such as pow(), sort(), abs(), and etc.Refer to Java API Documentation.
double x = Math.pow( 2, 3 ); //8.0
double y = Math.sqrt( 3.0 ); //1.732
int n = Math.abs( -12 ); //12
double y = Math.sqrt( 3.0 ); //1.732
int n = Math.abs( -12 ); //12
Scope
Variables have certain scopes which define their accessibility.Local variables are declared inside a method. They end when the method is ended.
Style, Format, and Documentation
Programs should use proper indentation, format, and documentations.Each block of code should be indented a few spaces within its block.
Blank lines should be used to separate sections.
Each program should have a header information to include the auther, date of completion, program name, and program description.