Repetition Structures / Loops
With loops, the program can execute a section of code repeatedly as long as the condition remains true.
In Chapter 4, we study the following concepts:
Increment(++) and Decrement(--) operators
while Loop
Using the while Loop for
Input Validation
Counter-Controlled while Loop
Sentinel-Controlled while Loop
Flag-Controlled while Loop
do … while Loop
for Loop
Nested Loop
break Statement
continue Statement
File Input Output (File IO) and Random Numbers
Increment and Decrement Operators
++ Increment by 1
-- Decrement by 1
-- Decrement by 1
int n = 5;
n++; //Equivalent to n = n + 1
n--; //Equivalent to n = n - 1
Prefix ( ++n) vs. postfix ( n++ )n++; //Equivalent to n = n + 1
n--; //Equivalent to n = n - 1
When they are used independently, the effect is the same.
The difference is when they are used in an expression.
int x, y;
x = 5;
y = ++x; //increment x first, then use the new value of x to assign to y
System.out.println(x); //6 is printed.
System.out.println(y); //6 is printed.
x = 5;
y = ++x; //increment x first, then use the new value of x to assign to y
System.out.println(x); //6 is printed.
System.out.println(y); //6 is printed.
int x, y;
x = 5;
y = x++; //assign the current value of x to y first, then increment x by 1
System.out.println(x); //6 is printed.
System.out.println(y); //5 is printed.
x = 5;
y = x++; //assign the current value of x to y first, then increment x by 1
System.out.println(x); //6 is printed.
System.out.println(y); //5 is printed.
while Loop
The program can use a while loop to execute a section of code repeatedly as long as the conditions remains true.The general structure is shown below.
while (condition) { //condition - logical/boolean expression
Java Code
}
There are three tokens to control the loop execution.Java Code
}
n = 1; //Token 1: initialization of the control variable
while (n <= 100) { //Token 2: test the loop condition
System.out.println(n);
n++; //Token 3: change the control variable
}
while (n <= 100) { //Token 2: test the loop condition
System.out.println(n);
n++; //Token 3: change the control variable
}
As long as the while loop's condition is true,
the statements inside of the while loop body will execute.
The while loop is a pretest loop, so it will test the condition prior to execute the body of the loop.
The while loop's body may not execute at all if the condition is false initially.
It is very important to set the condition to false somewhere in the loop so it will terminate. Otherwise, the loop becomes an infinite loop.
The while loop is a pretest loop, so it will test the condition prior to execute the body of the loop.
The while loop's body may not execute at all if the condition is false initially.
It is very important to set the condition to false somewhere in the loop so it will terminate. Otherwise, the loop becomes an infinite loop.
Flowchart:
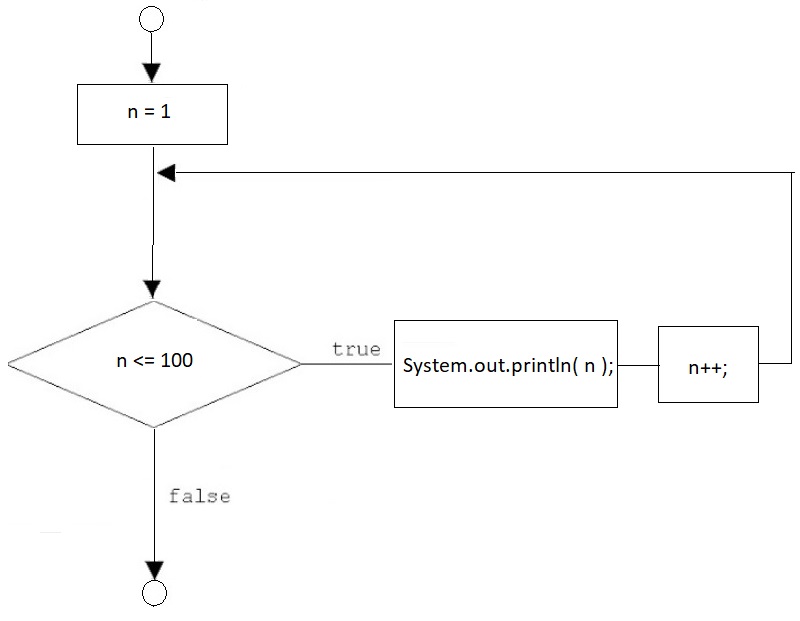
To make a decision, we use the same relational operators as in the selection structures.
Example:
n = 1;
while (n <= 100) {
System.out.println(n);
n++;
}
while (n <= 100) {
System.out.println(n);
n++;
}
n = 100;
while (n >= 1) {
System.out.println(n);
n--;
}
while (n >= 1) {
System.out.println(n);
n--;
}
n = 1;
while (n <= 100) {
System.out.println(n);
n += 5;
}
while (n <= 100) {
System.out.println(n);
n += 5;
}
Using the while Loop for Input Validation
Input validation is the process of ensuring that the user input is valid.
System.out.print("Enter a positive integer: ");
number = keyboard.nextInt();
while (number < 0) {
System.out.println("That number is invalid.");
System.out.print("Enter a a positive integer: ");
number = keyboard.nextInt();
}
number = keyboard.nextInt();
while (number < 0) {
System.out.println("That number is invalid.");
System.out.print("Enter a a positive integer: ");
number = keyboard.nextInt();
}
Counter-Controlled while Loop
We use a variable to control the number of iterations the loop should execute.Basically, we know in advance the number of iterations the while loop will execute.
//input 10 numbers and print the average value
int count = 0, n, sum = 0;
while ( count < 10 ) {
System.out.print("Enter an integer: ");
n = keyboard.nextInt();
sum += n;
count++;
}
System.out.println("Average: " + (double)sum / 10);
int count = 0, n, sum = 0;
while ( count < 10 ) {
System.out.print("Enter an integer: ");
n = keyboard.nextInt();
sum += n;
count++;
}
System.out.println("Average: " + (double)sum / 10);
Sentinel-Controlled while Loop
For some programming problems, we may not know how many iterations the while loop should execute.In this case, a special value called sentinel value is used to indicate the “end of loop”.
//input a number of integers and print the average value
int count = 0, n, sum = 0;
System.out.print("Enter an integer: ");
n = keyboard.nextInt();
while ( n != -1 ) { //-1 is the sentinel value
sum += n;
count++;
//repeat the input statement
System.out.print("Enter an integer: ");
n = keyboard.nextInt();
}
System.out.println("Average: " + (double)sum / count );
int count = 0, n, sum = 0;
System.out.print("Enter an integer: ");
n = keyboard.nextInt();
while ( n != -1 ) { //-1 is the sentinel value
sum += n;
count++;
//repeat the input statement
System.out.print("Enter an integer: ");
n = keyboard.nextInt();
}
System.out.println("Average: " + (double)sum / count );
For this section of code, the loop will be terminated when the input value is -1 (sentinel value). Otherwise, it will continue to execute.
Flag-Controlled while Loop
It is similar to the sentinel-controlled loop.A boolean value is used to control the loop execution.
//input a number of integers and print the average value
int count = 0, n, sum = 0;
boolean flag = true;
while ( flag ) { //The boolean value is used to control the loop.
System.out.print("Enter an integer: ");
n = keyboard.nextInt();
if( n == -1) {
flag = false; //set flag to false so the loop can terminate.
}
}
System.out.println("Average: " + (double)sum / count );
int count = 0, n, sum = 0;
boolean flag = true;
while ( flag ) { //The boolean value is used to control the loop.
System.out.print("Enter an integer: ");
n = keyboard.nextInt();
if( n == -1) {
flag = false; //set flag to false so the loop can terminate.
}
}
System.out.println("Average: " + (double)sum / count );
For this section of code, a boolean flag is used to control the loop.
Initially the flag is set to true so the loop will continue to execute.
When the input value is -1, the flag is set to false so the loop will be terminated.
do...while Loop
The do-while loop is a post-test loop, which means it will execute the loop prior to testing the condition.The general structure is shown below.
do {
statements;
} while ( logical expression );
statements;
} while ( logical expression );
Example:
int n = 1;
do {
System.out.println ( n );
n++;
} while (n <= 100);
do {
System.out.println ( n );
n++;
} while (n <= 100);
The loop body is executed first.
Then the condition is evaluated.
If condition is true, the loop body is executed again.
The loop terminates when the condition is false.
Then the condition is evaluated.
If condition is true, the loop body is executed again.
The loop terminates when the condition is false.
Flowchart:
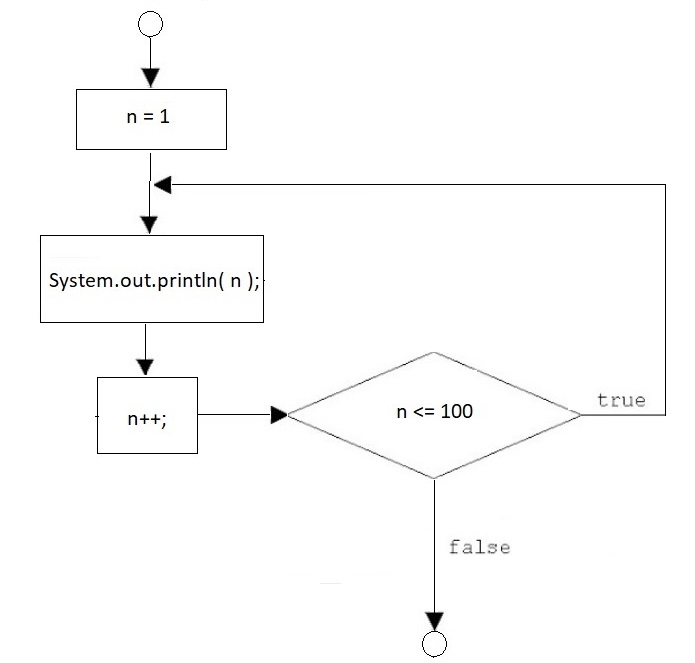
The difference between while and do-while loops:
The body of a do-while loop will execute at least once.
The body of a while loop may not execute at all.
The body of a while loop may not execute at all.
for Loop
With for loop, we group the three tokens on the same line.Basically, we initialize the control variable, test the condition, and modify the control variable on one line, separated by semi-colons.
It is generally used to handle counter-controlled repetition.
It is a pre-test loop.
The general structure is shown below.
for(initialization; test; update) {
statements;
}
statements;
}
Example:
for (int n = 0; n <= 100; n++) {
System.out.println(n);
}
System.out.println(n);
}
When the loop is executed, n is initialized to 0.
Then n is tested to see if it’s less than 100.
If true, the loop body is executed.
Afterwards, increment n by 1.
The loop body will execute as long as the condition is true.
Then n is tested to see if it’s less than 100.
If true, the loop body is executed.
Afterwards, increment n by 1.
The loop body will execute as long as the condition is true.
Flowchart:
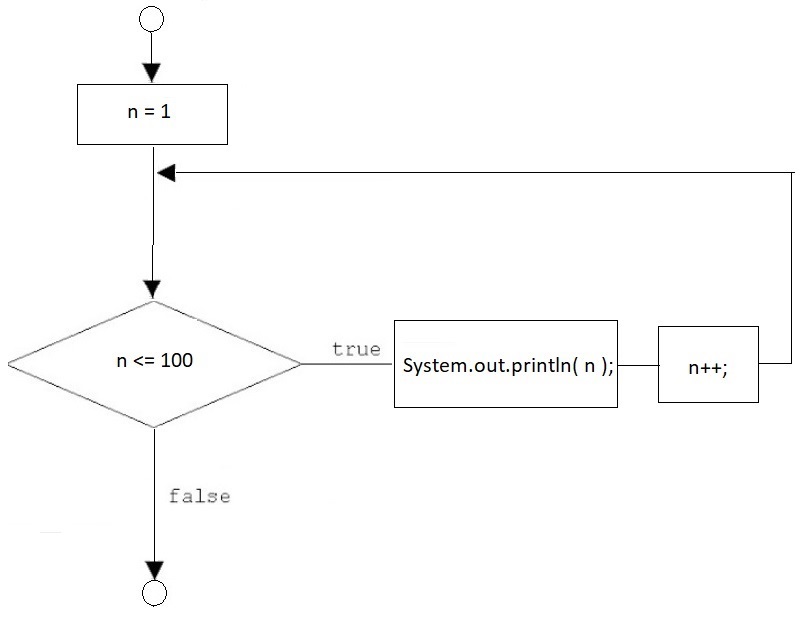
Important Notes with for Loop
Variables declared inside the for statement have local
scope which are only available for the for loop.
If logical expression is omitted, it is assumed to be true.
If the for statement ends with a semicolon, the for loop doesn't have a body.
If all three tokens are omitted, it is an infinite loop.
If logical expression is omitted, it is assumed to be true.
If the for statement ends with a semicolon, the for loop doesn't have a body.
If all three tokens are omitted, it is an infinite loop.
for( ; ; ) { }
We should not update the control variable within the body of the
loop. It can make debugging difficult.
Nested Loop
Like if statements, loops can be nested.A nested loop is one loop inside another loop.
If a loop needs to be repeated more than once, we can use a nested loop.
If a loop is nested, the inner loop will execute all of its iterations for each outer loop iteration.
We can use nested loops to process data in rows and columns.
The inner loop handles the columns and the outer loop handles the rows.
Example:
for( i = 0; i<10; i++ ) {
printf(“\n”);
}
for ( j = 0; j<10; j++) {
print( "* " );
}
print( "* " );
}
printf(“\n”);
}
This example prints a 10 x 10 square of *.
break Statement
With in a loop or switch, the break statement causes an immediate exit from the loop or the switch.It is often used to terminate a loop.
However, the use of the break statement in loops can make code hard to maintain because it bypasses the normal execution sequence.
Example:
int i;
for ( i = 0; i<100; i++) {
System.out.println( i );
if (i == 10) {
break;
}
}
for ( i = 0; i<100; i++) {
System.out.println( i );
if (i == 10) {
break;
}
}
This example prints 0 to 10.
continue Statements
With in a loop, the continue statement skips the remaining code in the body of loop and performs the next iteration.It can make the program difficult to debug.
Example:
for(int x = 0; x < 10; x++) {
if(x == 5) {
continue;
}
System.out.println(" " + x );
}
This example prints 0 1 2 3 4 6 7 8 9.