OOP - Encapsulation
In Chapter 6 and Chapter 8 (Skip Section 8.7 - Aggregation), we study Object Oriented Programming (OOP).
Introduction to OOP
Objects
Classes
Encapsulation
Two Steps to Write OOP Program
Class Definition and UML Diagram
Information Hiding
Constructors
Setters and Getters
Instance Variables and Methods
toString Method
RightTriangle Class Definition
Creating Objects to Solve Problems
in the Client Program
Reserved keyword “this” Instance Variables & Methods vs. Static variables & Static Methods Comparing Objects Changing Private Data Members Shallow Copy vs. Deep Copy
Reserved keyword “this” Instance Variables & Methods vs. Static variables & Static Methods Comparing Objects Changing Private Data Members Shallow Copy vs. Deep Copy
Introduction to OOP
So far, we have been using Procedural Programming approach to write programs.Transition from Procedural Programming to OOP is not always smooth.
OOP - Object Oriented Programming is to use interactive objects to solve problems.
Objects
The following table shows several real-life objects.Each object has a set of attributes and functions.
Attributes define the object's properties.
i.e. Two different vehicles have different attributes such as model, color, price, and etc.
Functions are the behaviors and they are the tasks the objects are supposed to perform.
i.e. Two different vehicles have different attributes such as model, color, price, and etc.
Functions are the behaviors and they are the tasks the objects are supposed to perform.
Objects | Attributes / Properties | Functions / Behaviors |
---|---|---|
Vehicle | model, year, color price …. | drive forward, reverse, brake … |
Door | length, width, material …. | open, close … |
Employee | name, title, email, salary … | work, earning … |
Bank Account | account number, name, balance …. | deposit, withdraw …. |
Classes
A class is a data type. This type of data type is called abstract data type (ADT).An ADT is a data type whose behavior is defined by its data members and methods.
In OOP, we first create the new data type (ADT). Later we can create objects out of the class to solve the problem.
The following table shows the classes for the objects described above.
The object's attributes are data members. They are basically variables.
The functions are the methods that perform tasks.
Classes | Data Members / Instance Variables | Instance Methods |
---|---|---|
Vehicle | model, year, color price …. | drive forward, reverse, brake … |
Door | length, width, material …. | open, close … |
Employee | name, title, email, salary … | work, earning … |
Bank Account | account number, name, balance …. | deposit, withdraw …. |
Encapsulation
We combine both data members (variables) and methods into the class. It is called encapsulation.Example:
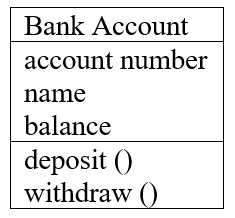
Two steps to write OOP program
Step 1: Define the class (write ADT)
Step 2: Create objects out of the class to solve the problem
Step 2: Create objects out of the class to solve the problem
Class Definition and UML Diagram
Let’s design the RightTriangle class.A right triangle has two attributes: side 1 and side 1.
Its functions include calculating side 3, area, and perimeter.
We use UML (Unified Modeling Language) notations to represent the class RightTriangle.
It is called the class diagram.
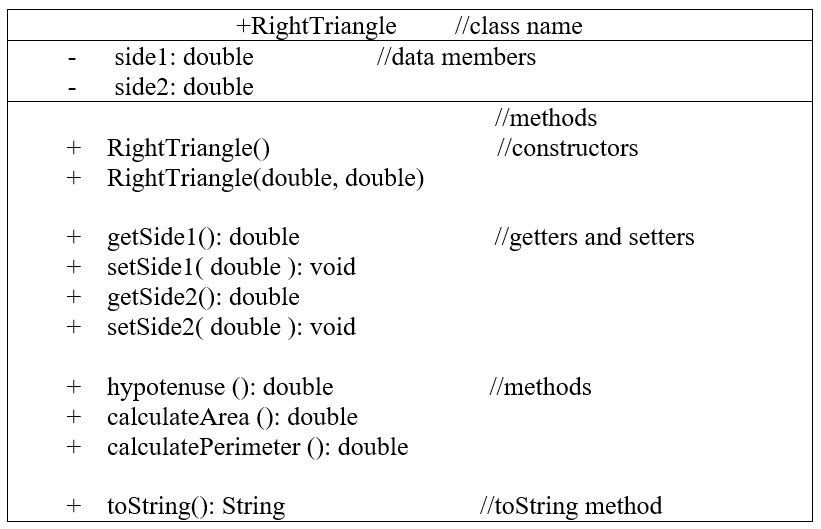
Access modifiers determine the members accessibility.
There are four access modifiers:
- private (only accessible within the class)
+ public (accessible outside the class)
# protected (accessible by the subclasses and classes within the same package)
default (accessible by the classess within the same package)
In COMP 2243, we study two of them, private and public.
Information Hiding
All the data members should be private.Data members as public, protected, and default are not acceptable in COMP 2243.
Due to the fact that the private data members are not accessible outside the class, we can use the private access modifier to hide the data members within the class and control their access.
Information hiding helps to ensure object's data integrity.
Classes are typically used as components in software development.
Methods should be public so they can be used to access and manipulate the private data members.
Classes are typically used as components in software development.
Methods should be public so they can be used to access and manipulate the private data members.
Constructors
Constructors are special methods.
The purpose of the constructor is to create object and to
initialize the data members to certain values that reflect
the state of the object.
Constructors have the same name as the class.
Constructors have no return type (not even void).
Constructors may not return any values.
Constructors are public.
Constructors can be overloaded, so a class can have more than one constructor. All of them have the same name, but different signatures (parameters).
Constructors have the same name as the class.
Constructors have no return type (not even void).
Constructors may not return any values.
Constructors are public.
Constructors can be overloaded, so a class can have more than one constructor. All of them have the same name, but different signatures (parameters).
Default constructor
If you do not write a constructor, Java provides a default constructor
when the class is compiled.
The default constructor is a constructor with no parameters and used to initialize an object to the default values.
The default constructor is a constructor with no parameters and used to initialize an object to the default values.
Numeric data members: 0
Boolean data members: false
Reference data members: null
Boolean data members: false
Reference data members: null
Getters and Setters
They are get and set methods, also called accessors and mutators.For each private data member, typically, we provide a pair of public getter and setter.
Getter – retrieve private data
Setter – change private data
Setter – change private data
Instance Variables and Methods
Every object has its own copy of instance variables.Instance methods are methods that are not declared with a special keyword, static.
For instance variables and instance methods, it is required
to create objects and then use object references to use them.
Basically you have to create objects to use them. Otherwise, they simply do not exist.
Basically you have to create objects to use them. Otherwise, they simply do not exist.
toString Method
Whenever we create an object, the Java provides a default toString() method.toString() method is used to convert an object to a String object.
It returns a string representation of the object.
Basically, we need to format the data members appporiately and reutrn the string representation.
When we print the object, toString() method is called.
The default toString() method returns a string that is the name of the class, followed by the hash code of the object.
We should always override it.
public String toString() { //signature of toString()
}
}
RightTriangle Class Definition
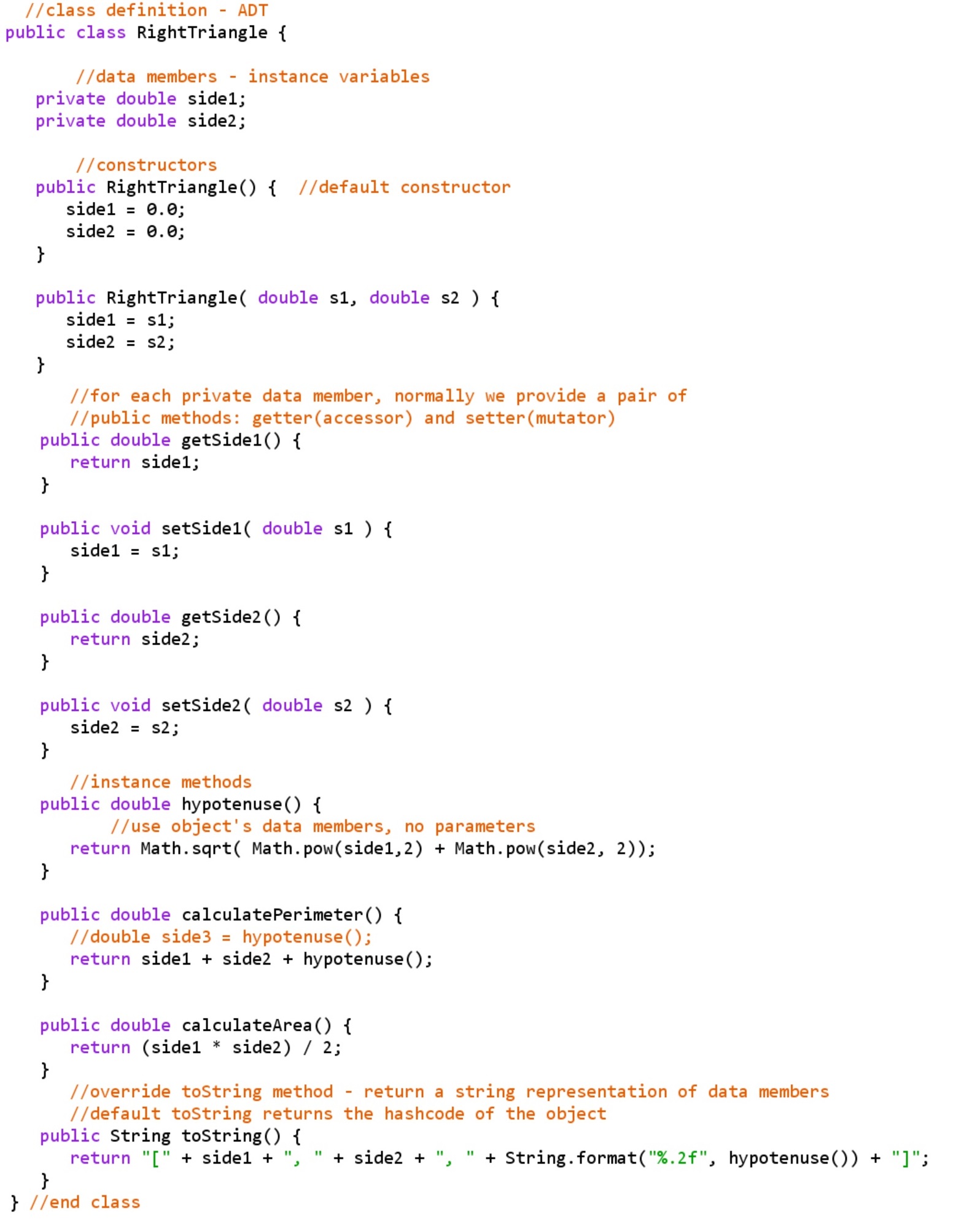
Creating Objects to Solve Problems in the Client Program
Once the class definition is completed, we can create objects in the client program and use these objects to solve problems.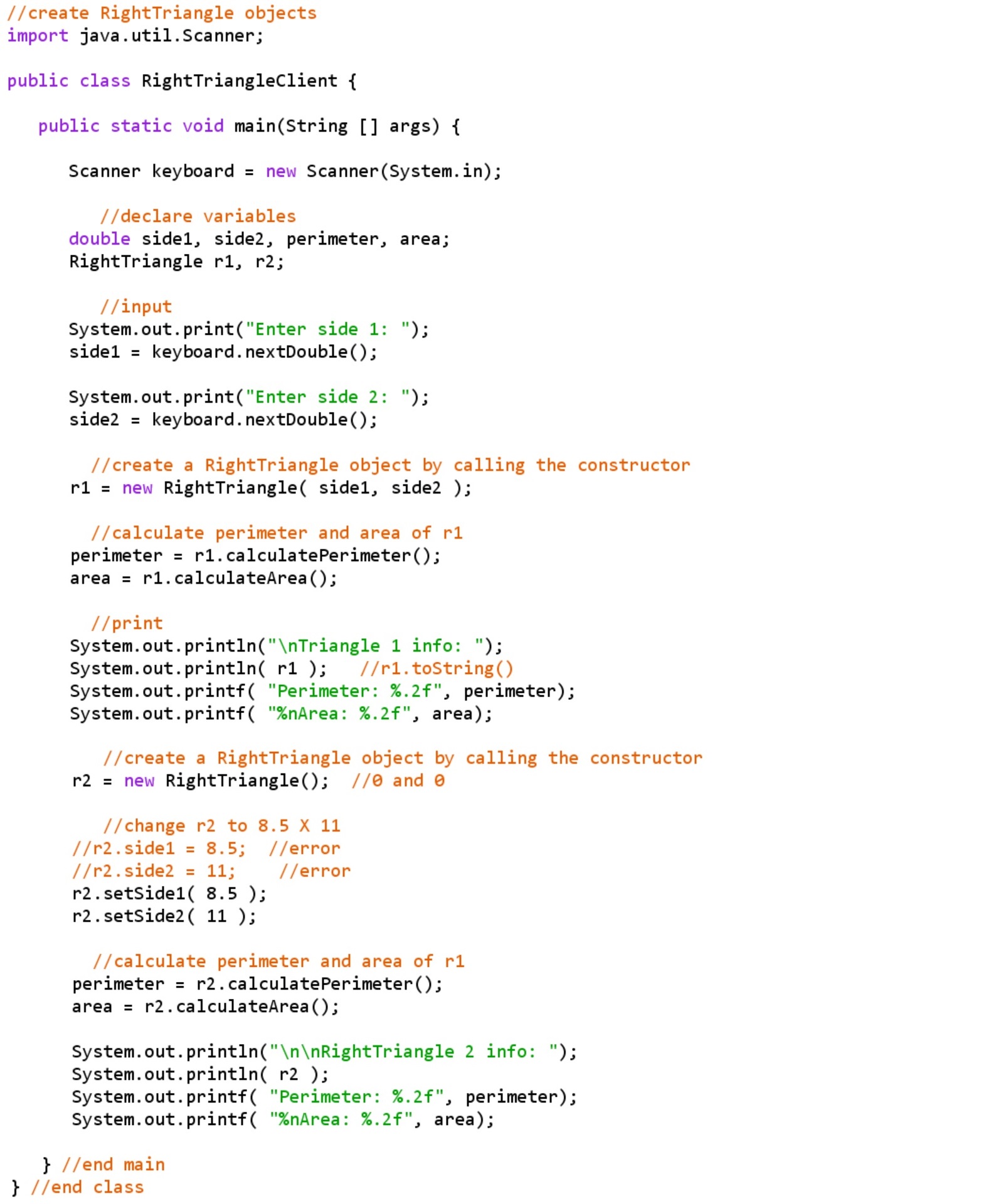
Reserved Keyword "this"
“this” is called a self-referencing pointer.It refers to the object itself.
If the instance variable and the parameter of constructor or method have the same name, the parameter creates a shadow to make the instance variable invisible in the constructors or methods.
We can use "this" reference to overcome shadowing.
We can also use "this" reference to call a constructor from another constructor.
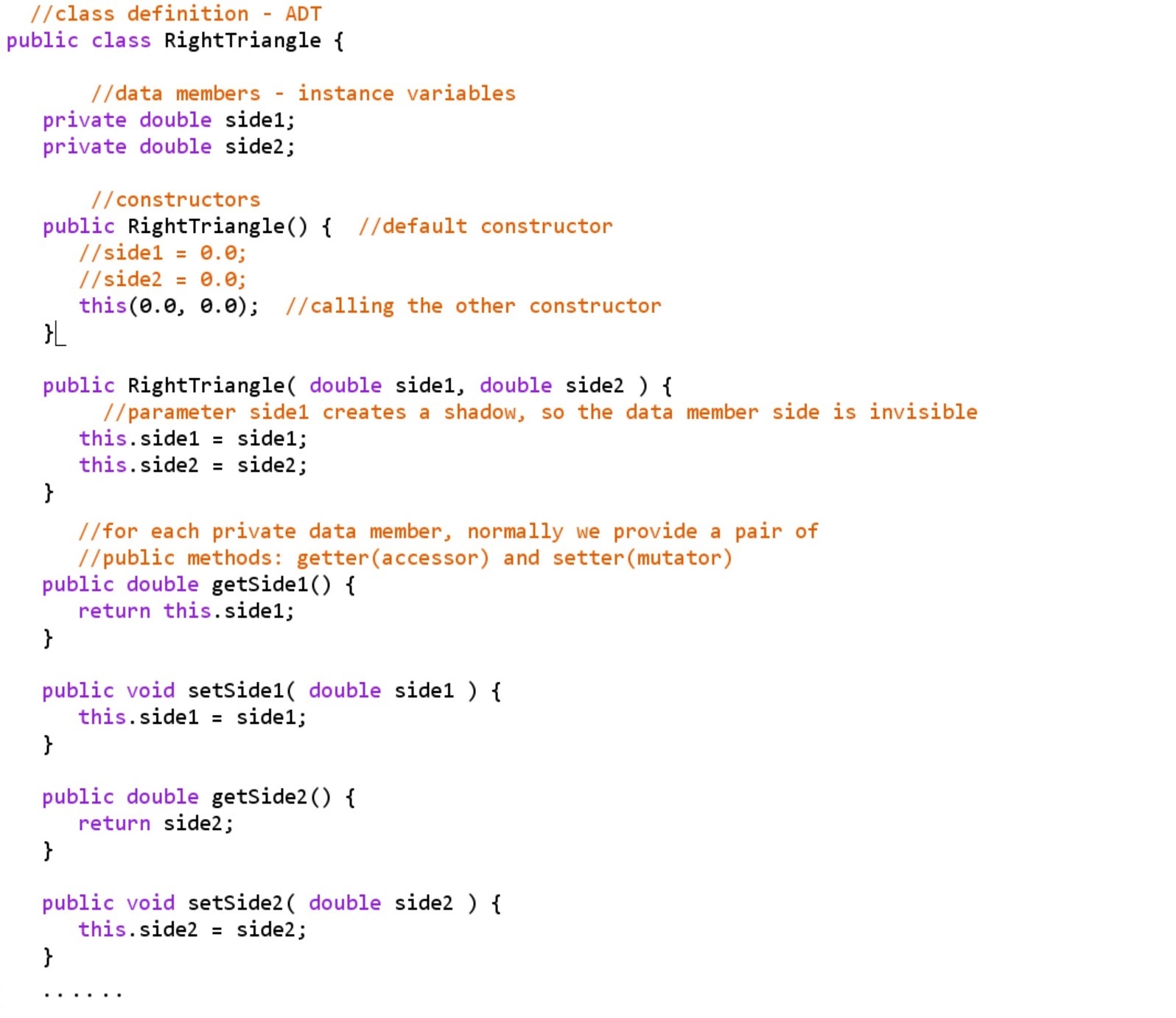
Instance Variables & Methods vs. Static variables & Static Methods
Instance variables
Every object (instance) has its own copy of the instance variables.
They simply do not exist without any object.
They simply do not exist without any object.
Static variables
They are class wide variables that are shared by every object of the same class.
They apply to the entire class.
They exist even before any object is being created.
They apply to the entire class.
They exist even before any object is being created.
Instance methods
They belong to objects.
We must create an object first, then use the object reference to call the method.
Example:
We must create an object first, then use the object reference to call the method.
Example:
RightTriangle r1 = new RightTriangle( 12.3, 23.45 ); //create the object first
System.out.println( r1.calculatePerimeter() ); //use object reference to call instance method
System.out.println( r1.calculatePerimeter() ); //use object reference to call instance method
Static methods
They exist without any object.
We don’t need to create any objects to use the static methods.
We don’t need to create any objects to use the static methods.
Example:
Math.pow( 2, 3 ); //use class name to call the static method
Comparing Objects
When comparing two objects with the == operator, the memory address of the objects are compared.The contents of the objects are not compared.
We cannot compare two objects with <, <=, >, or >= operators.
Instead, we need to specify what field to compare.
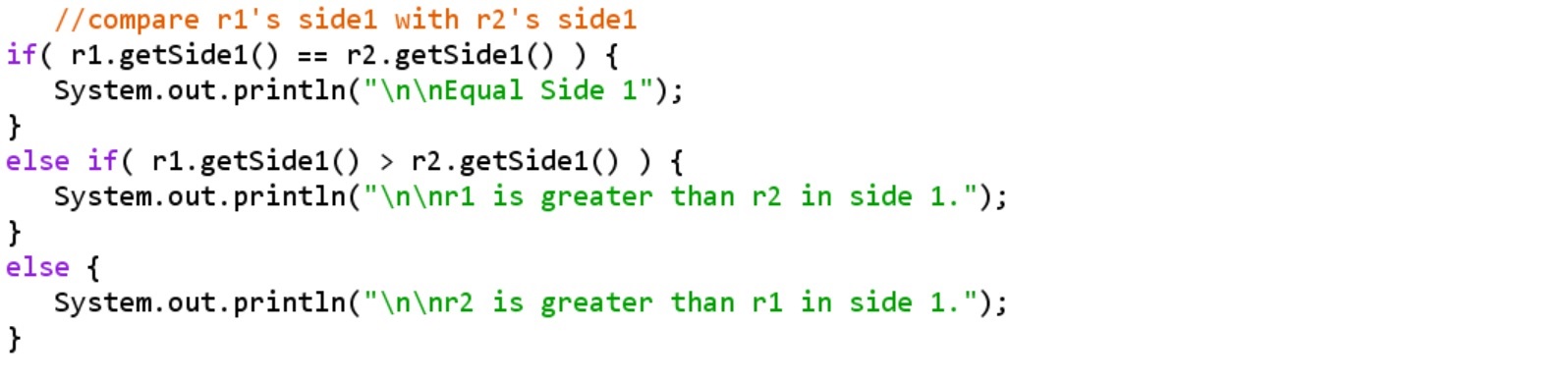
All objects have an equals method.
The default operation of the equals method is to compare memory addresses of the objects (just like the == operator).
We can override it.
Changing Private Data Members
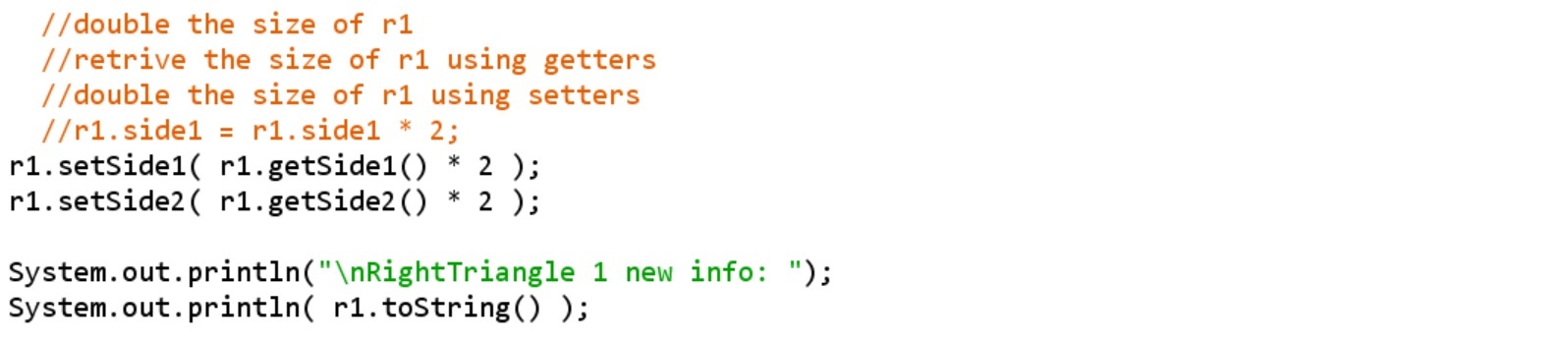
Shallow Copy vs. Deep Copy
There are two ways to copy an object: shallow copy and deep copy.Shallow copy:
use = operator to assign an object reference to another reference.
This is simply copying the address of an object into another reference variable.
Essentially, one single object has two references.
Create a new object first
Copy the data members from one object into the new object
We can use either a copy method or a copy constructor for deep copying.
The following example is to copy Fraction objects.
The Fraction object has two data members: numerator and denominator.
The copy constructor assigns "other" object's data members to "this" new object.
The copy method returns a new object based on the current object ("this" object).
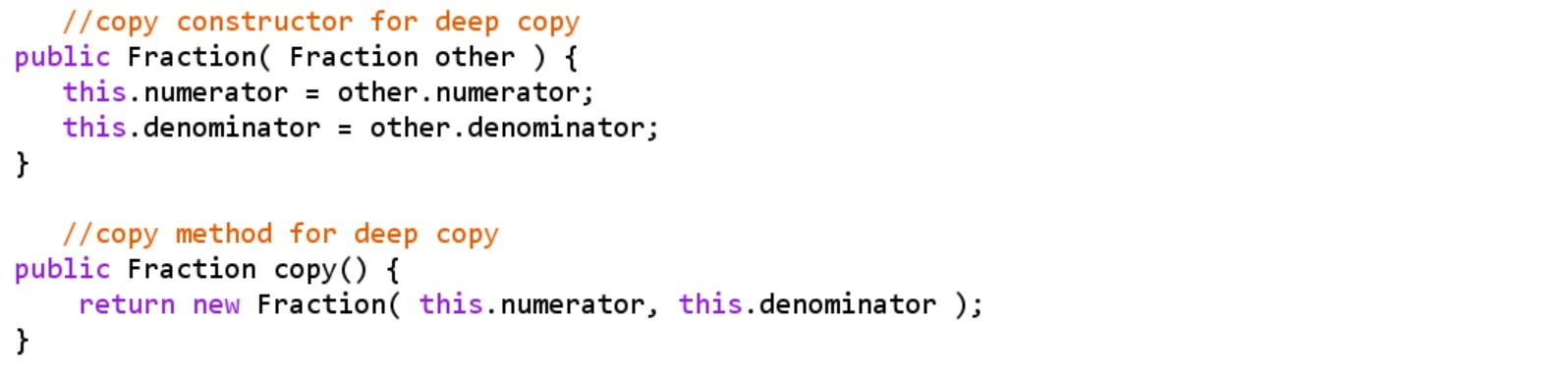