Text Processing
In Chapter 9, we study:
Wrapper Classes
There are eight primitive data types. They are called “primitive” because they are not created from classes.For each primitive data type, Java provides a corresponding wrapper class.
byte - Byte
short - Short
int - Integer
long - Long
float - FLoat
double - Double
boolean - Boolean
short - Short
int - Integer
long - Long
float - FLoat
double - Double
boolean - Boolean
A wrapper class is a class that is “wrapped around” a primitive data type.
The wrapper classes are part of java.lang package, so there is no import statement required.
Wrapper classes allow you to create objects to represent primitives.
Wrapper classes are immutable, which means that once we created an object, we cannot change the object’s value.
Wrapper classes provide static methods that are very useful.
Objects of wrapper classes and primitive data values can be used interchangeably due to auto boxing and auto unboxing.
Integer n1 = 25; //auto boxing
int k = n1; //auto unboxing
int k = n1; //auto unboxing
Character Class
The Character class allows a char data type to be wrapped in an object.The Character class provides methods that can test, process, and convert character data.
Some examples of Character methods:
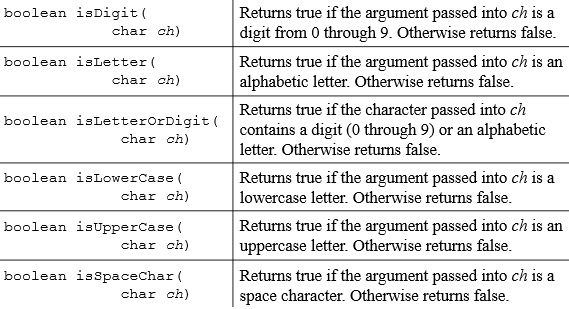
Refer to Java API Documentation for more Character methods.
String Class
String objects are immutable. Once the object is created, we cannot change it.String class has methods to manipulate string objects, but they do not change the original string objects.
Instead, these methods return the manipulated string objects.
String name = “Tom”;
name = name.toUpperCase(); //return uppercase TOM
name = name.toUpperCase(); //return uppercase TOM
We have studied several important string methods in the "Fundamentals" section.
Refer to Java API Documentation for more String methods.
StringBuilder Class
The StringBuilder class is similar to the String class.But, StringBuilder objects are mutable. We can change the contents of StringBuilder objects.
For example, we can change specific characters, insert characters, delete characters, and etc.
A StringBuilder object can grow or shrink in size, as needed, to accommodate the changes.