Decision / Selection Structures
In general, a program can be written with three structures:
Sequence Structure: - executing a program in sequence
Decision Structure: – making a decision and executing a section of code based the decision
Repetition Structure: – executing a section of code repeatedly using a loop
Decision Structure: – making a decision and executing a section of code based the decision
Repetition Structure: – executing a section of code repeatedly using a loop
In Chapter 3, we study the following concepts:
The if Statement
The if-else Statement
The nested if Statement
The if-else-if Statement
The Logical Operators
Comparing String Objects
The Conditional Operator
The switch Statement
Formatting the output with printf
and String.format methods
The if Statement
A program can use the if statement to make a decision based on a condition / logical expression.The condition returns a boolean value, either truth or false.
If the condition is true, the statement in the body of the if statement is executed.
This is called one-way selection structure.
if (condition) {
Java Code
}
To make a decision, we use one of six relational operators:Java Code
}
== equal to
!= not equal to
< less than
<= less than or equal to
> greater than
>= greater than or equal to
The expression with relational operator is call logical expression. != not equal to
< less than
<= less than or equal to
> greater than
>= greater than or equal to
It returns either true or false.
Example:
//n is a positive integer.
if (n < 10) {
System.out.println(“n is a single digit number.”);
}
if (n < 10) {
System.out.println(“n is a single digit number.”);
}
//n1, n2, and n3 are integers.
if(n2 != 0) {
n3 = n1 / n2; //ensure n2 is not 0 before performing division operation.
}
if(n2 != 0) {
n3 = n1 / n2; //ensure n2 is not 0 before performing division operation.
}
//n is an integer.
if(n < 0) {
n = -n; //absolute value
}
Flowchart:if(n < 0) {
n = -n; //absolute value
}
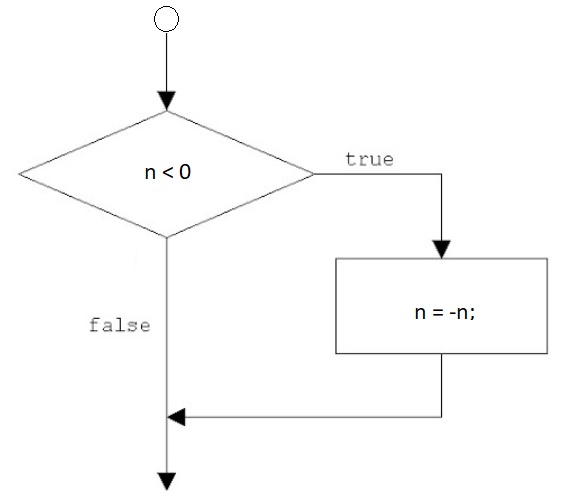
The if-else Statement
With the if-else statement, the program can make a decision to determine which section of code to execute among two sections of code.
if (condition) {
Java Code Block 1
}
else {
Java Code Block 2
}
If the condition is true, the block 1 is executed;Java Code Block 1
}
else {
Java Code Block 2
}
If the expression is false, the block 2 is executed.
This is called two-way selection structure.
Example:
//n1 and n2 are integers.
if(n2 != 0) {
n3 = n1 / n2; //ensure n2 is not 0 before performing division operation.
}
else {
System.out.println("Error, n2 must be a non-zero integer.");
}
if(n2 != 0) {
n3 = n1 / n2; //ensure n2 is not 0 before performing division operation.
}
else {
System.out.println("Error, n2 must be a non-zero integer.");
}
Flowchart:
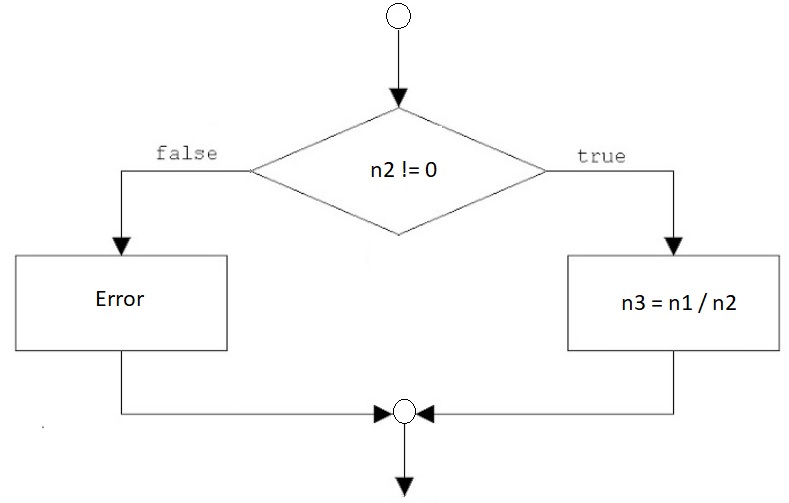
The nested if Statement
With the nested if statement, an if statement can appear inside another if statement or else statement.Likewise, an if-else statement can appear inside another if statement or else statement.
Example:
//n is an integer.
if(n <=0) {
System.out.println("Error, n must be greater than 0.");
}
else {
if(n < 10) {
System.out.println("n is a single-digit integer.");
}
else {
System.out.println("n has two or more digits.");
}
}
if(n <=0) {
System.out.println("Error, n must be greater than 0.");
}
else {
if(n < 10) {
System.out.println("n is a single-digit integer.");
}
else {
System.out.println("n has two or more digits.");
}
}
The if-else-if Statement
The nested if statements can get quite complicated.The if-else-if statement makes nested if decision logic simpler to write.
This is called multiple selection structure.
Example:
//score is between 0 and 100
if(score >= 90) {
System.out.println("A");
}
else if(score >= 80) {
System.out.println("B");
}
else if(score >= 70) {
System.out.println("C");
}
else if(score >= 60) {
System.out.println("D");
}
else {
System.out.println("F");
}
if(score >= 90) {
System.out.println("A");
}
else if(score >= 80) {
System.out.println("B");
}
else if(score >= 70) {
System.out.println("C");
}
else if(score >= 60) {
System.out.println("D");
}
else {
System.out.println("F");
}
This example contains a single multiple selection structure. Therefore, if one condition clause is met, the other condition clauses are skipped.
The Logical Operators
With the logical (Boolean) operators, we can combine multiple logical expressions.The are two binary logical operators: && and || , and one unary logical operator: !.
&& AND:
Combine two boolean expressions into one.
Both expressions must be true for the overall expression to be true.
|| OR:
Combine two boolean expressions into one.
Only one expression must be true for the overall expression to be true.
! NOT:
It reverses a boolean expression's logic.
Truth Table of Logical Operators:Combine two boolean expressions into one.
Both expressions must be true for the overall expression to be true.
|| OR:
Combine two boolean expressions into one.
Only one expression must be true for the overall expression to be true.
! NOT:
It reverses a boolean expression's logic.
Exp1 | Exp2 | Exp1 && Exp2 |
---|---|---|
False | False | False |
False | True | False |
True | False | False |
True | True | True |
Exp1 | Exp2 | Exp1 || Exp2 |
---|---|---|
False | False | False |
False | True | True |
True | False | True |
True | True | True |
Exp | !Exp |
---|---|
False | True |
True | False |
if(length > 0 && width > 0) {
area = length * width; //ensure both length and width are valid data.
}
else {
System.out.println("Error, Invalid Data.");
}
area = length * width; //ensure both length and width are valid data.
}
else {
System.out.println("Error, Invalid Data.");
}
Operator Precedence:
Order of Precedence | Operator |
---|---|
1 | ! |
2 | * / % |
3 | + - |
4 | < <= > >= |
5 | == != |
6 | && |
7 | || |
8 | = += -= *= /= %= |
Comparing String Objects
A reference variable contains the address of the object.Unless two references refer to the same object, the relational operator (==) will not return true if two references are compared.
To compare the contents of two String objects, do not use the relational operators.
Example:
System.out.print("Enter a string for s1: ");
s1 = keyboard.next();
System.out.print("Enter a string for s2: ");
s2 = keyboard.next();
//assume the user entered the same string for s1 and s2: Hello
if(s1 == s2) { //The condition is false, because s1 and s2 refer to different objects.
System.out.println("\n" + s1 + " is equal to " + s2 + ".");
}
else {
System.out.println("\n" + s1 + " is not equal to " + s2 + ".");
}
s1 = keyboard.next();
System.out.print("Enter a string for s2: ");
s2 = keyboard.next();
//assume the user entered the same string for s1 and s2: Hello
if(s1 == s2) { //The condition is false, because s1 and s2 refer to different objects.
System.out.println("\n" + s1 + " is equal to " + s2 + ".");
}
else {
System.out.println("\n" + s1 + " is not equal to " + s2 + ".");
}
How to compare String objects?
There are four string methods for comparing string objects.
equals
equalsIgnoreCase
compareTo
compareToIgnoreCase
equalsIgnoreCase
compareTo
compareToIgnoreCase
equals and equalsIgnoreCase methods compare the equality of two string objects, and return true or false.
System.out.print("Enter a string for s1: ");
s1 = keyboard.next();
System.out.print("Enter a string for s2: ");
s2 = keyboard.next();
//assume the user entered the same string for s1 and s2: Hello
if(s1.equals(s2)) { //The condition is true.
System.out.println("\n" + s1 + " is equal to " + s2 + ".");
}
else {
System.out.println("\n" + s1 + " is not equal to " + s2 + ".");
}
s1 = keyboard.next();
System.out.print("Enter a string for s2: ");
s2 = keyboard.next();
//assume the user entered the same string for s1 and s2: Hello
if(s1.equals(s2)) { //The condition is true.
System.out.println("\n" + s1 + " is equal to " + s2 + ".");
}
else {
System.out.println("\n" + s1 + " is not equal to " + s2 + ".");
}
compareTo and compareToIgnoreCase methods compare the unicode of two string objects one character at a time, and return an integer value.
System.out.print("Enter a string for s1: ");
s1 = keyboard.next();
System.out.print("Enter a string for s2: ");
s2 = keyboard.next();
if(s1.compareTo(s2) == 0) {
System.out.println("\n" + s1 + " is equal to " + s2 + ".");
}
else if (s1.compareTo(s2) < 0){
System.out.println("\n" + s1 + " is less than " + s2 + ".");
} else {
System.out.println("\n" + s1 + " is greater than " + s2 + ".");
}
s1 = keyboard.next();
System.out.print("Enter a string for s2: ");
s2 = keyboard.next();
if(s1.compareTo(s2) == 0) {
System.out.println("\n" + s1 + " is equal to " + s2 + ".");
}
else if (s1.compareTo(s2) < 0){
System.out.println("\n" + s1 + " is less than " + s2 + ".");
} else {
System.out.println("\n" + s1 + " is greater than " + s2 + ".");
}
If the returned value is 0, then both strings have the same
contents.
If the returned value is less than 0, then s1's unicode value is less than s2.
If the returned value is greater than 0, then s1's unicode value is greater than s2.
Note:
Example:
"Hello" and "Hi": e is less than i, so "Hello" is less than "Hi".
If the returned value is less than 0, then s1's unicode value is less than s2.
If the returned value is greater than 0, then s1's unicode value is greater than s2.
Note:
Characters in both strings are compared one pair at time.
If they are not equal, the comparison stops immediately.
If they are not equal, the comparison stops immediately.
Example:
"Hello" and "Hi": e is less than i, so "Hello" is less than "Hi".
The Conditional Operator
We can use the conditional operator ( ? : ) to write a simple statement that works like an if-else statement.Example:
x = 5;
y = 8;
n = x > y ? 1 : 2;
y = 8;
n = x > y ? 1 : 2;
Equivalent:
if (x > y) {
n = 1;
}
else {
n = 2;
}
n = 1;
}
else {
n = 2;
}
The switch Statement
The switch statement can evaluate an integer type, character type, or String type variable and make decisions based on the value.
A variable or expression is evaluated and different actions are
performed based on the evaluation.
The result of expression cannot be a boolean value.
It consists of a series of case labels and an optional default case.
The matching case code is executed.
The break statement ends the case statement.
If a case does not contain a break statement, then program execution continues into the next case.
The default case is to be executed if there is no matching case.
The default case is optional, but it is a good programming practice to include a default case.
The result of expression cannot be a boolean value.
It consists of a series of case labels and an optional default case.
The matching case code is executed.
The break statement ends the case statement.
If a case does not contain a break statement, then program execution continues into the next case.
The default case is to be executed if there is no matching case.
The default case is optional, but it is a good programming practice to include a default case.
Example:
System.out.print("Enter an integer: ");
k = keyboard.nextInt();
switch (k) {
case 1:
System.out.println("Action 1!");
break;
case 2:
System.out.println("Action 2!");
break;
case 3:
System.out.println("Action 3!");
break;
default:
System.out.println("No Action!");
}
k = keyboard.nextInt();
switch (k) {
case 1:
System.out.println("Action 1!");
break;
case 2:
System.out.println("Action 2!");
break;
case 3:
System.out.println("Action 3!");
break;
default:
System.out.println("No Action!");
}
System.out.printf and String.format methods
The System.out.printf MethodWe use this method to format output.
The general format of the method is:
System.out.printf( String object with place holders, argument list);
Format specifier / place holder:
%f --- decimal number
%d --- integral
%s --- string
%e --- scientific notation
%n --- new line
%d --- integral
%s --- string
%e --- scientific notation
%n --- new line
Example:
int number = 12389078;
String name = "Some Property";
double value = 568908.89;
double rate = 12.5; //12.5%
System.out.printf("Output: %n %n %,-13d %-16s %,-12.2f %-5.2f %s", number, name, value, rate, "%");
String name = "Some Property";
double value = 568908.89;
double rate = 12.5; //12.5%
System.out.printf("Output: %n %n %,-13d %-16s %,-12.2f %-5.2f %s", number, name, value, rate, "%");
The String.format Method
We use this method to format string object which can be printed later.
The general format of the method is:
String.format( String object with place holders, argument list);
The format specifiers / place holders are the same as
System.out.printf method.Example:
int number = 12389078;
String name = "Some Property";
double value = 568908.89;
double rate = 12.5; //12.5%
String result = String.format("Output: %n%n %,-13d %-16s %,-12.2f %-5.2f%s",
number, name, value, rate, "%");
JOptionPane.showMessageDialog( null, result );
String name = "Some Property";
double value = 568908.89;
double rate = 12.5; //12.5%
String result = String.format("Output: %n%n %,-13d %-16s %,-12.2f %-5.2f%s",
number, name, value, rate, "%");
JOptionPane.showMessageDialog( null, result );