ArrayProcessing Program
Description
This program creates an array of int to store 25 integers and then uses several methods to do the following:
Fill in the array with random numbers
Calculate the total value of the array elements
Find the smallest element in the array
Search a particular element in the array
Sort the array
Calculate the total value of the array elements
Find the smallest element in the array
Search a particular element in the array
Sort the array
Associated Concepts:
Array
Random number
Sequential search algorithm
Selection sort algorithm
Random number
Sequential search algorithm
Selection sort algorithm
Algorithm:
main method:
populateArray method definition:
printArray method definition:
sumArray method definition:
indexOfSmallest method definition:
sequentialSearch method definition:
selectionSort method definition:
Create an array of int to store 25 integers
Call methods to:
Call methods to:
Populate the array with random integers
Print array elements
Calculate the total value of the array elements
Find the index of the smallest element and print the smallest element
Search an element and print its index if found
Sort the array
Print the array elements in sorted order
Print array elements
Calculate the total value of the array elements
Find the index of the smallest element and print the smallest element
Search an element and print its index if found
Sort the array
Print the array elements in sorted order
populateArray method definition:
Create a Random object
For i = 0 to last index of array cell
For i = 0 to last index of array cell
Generate a random integer and assign it to cell i
printArray method definition:
For i = 0 to last index of array cell
Print the array element at i
sumArray method definition:
Declare variable sum and initialize it to 0
For i = 0 to last index of array cell
return sum
For i = 0 to last index of array cell
Add the current element at i to sum
return sum
indexOfSmallest method definition:
Declare variable minIndex and assign it to 0
(Assume the first element is the smallest)
For i = 1 to last index of array cell
return minIndex
For i = 1 to last index of array cell
If the current element at i is less than the
current smallest element
Update minIndex to the current index i
Update minIndex to the current index i
return minIndex
sequentialSearch method definition:
For i = 0 to last index of array cell
If the loop ended without returning i
Not found and return -1
If the current element at i is equal to the searched key
Found it and return i
Found it and return i
If the loop ended without returning i
Not found and return -1
selectionSort method definition:
Find the smallest element and swap it with the first element
Find the second smallest element and swap it with the second element
Continue the process ......
Find the second smallest element and swap it with the second element
Continue the process ......
Source Code
Download the source code file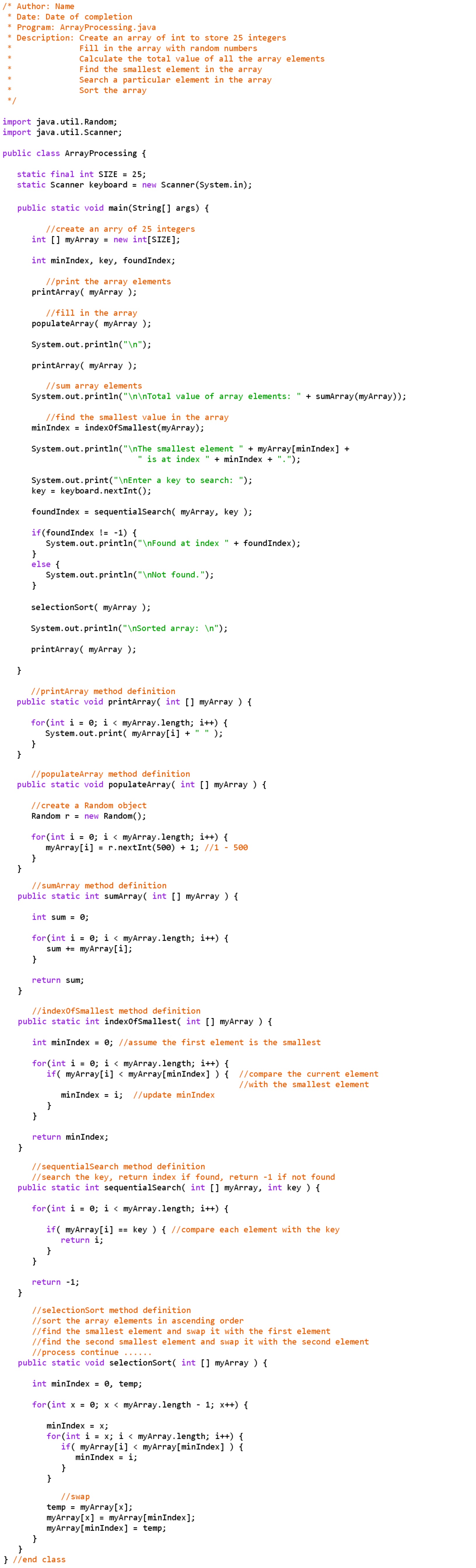
Sample Run
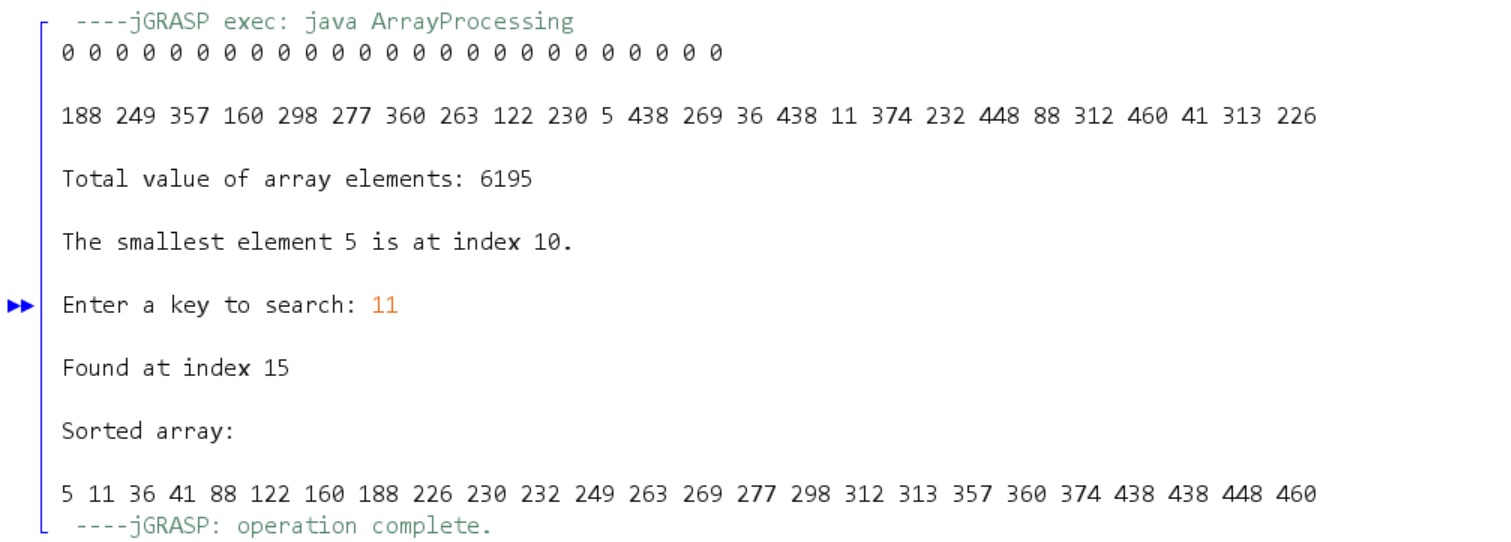