Hardware Store Program
Description
The program defines a class Inventory to represent hardware store product and uses an array to organize Inventory objects.The program reads an external data file which contains the inventory data to populate the array.
The users can print the inventory, search a particular product, calculate the total value of inventory, and etc.
Define Inventory class
Read external data file to fill in the array with Inventory objects
Print the inventory
Calculate the value of total inventory
Find the inventory with the highest value
Search a particular inventory in the array
Read external data file to fill in the array with Inventory objects
Print the inventory
Calculate the value of total inventory
Find the inventory with the highest value
Search a particular inventory in the array
This problem was originally presented as an exercise in C++ HOW TO PROGRAM (2nd Edition) book.
Exercise 14.12 (Page 738). Authors: Deitel & Deitel
The requirements are revised to meet our purpose.
Associated Concepts:
COMP2243 materials
Algorithm:
Inventory class diagram:
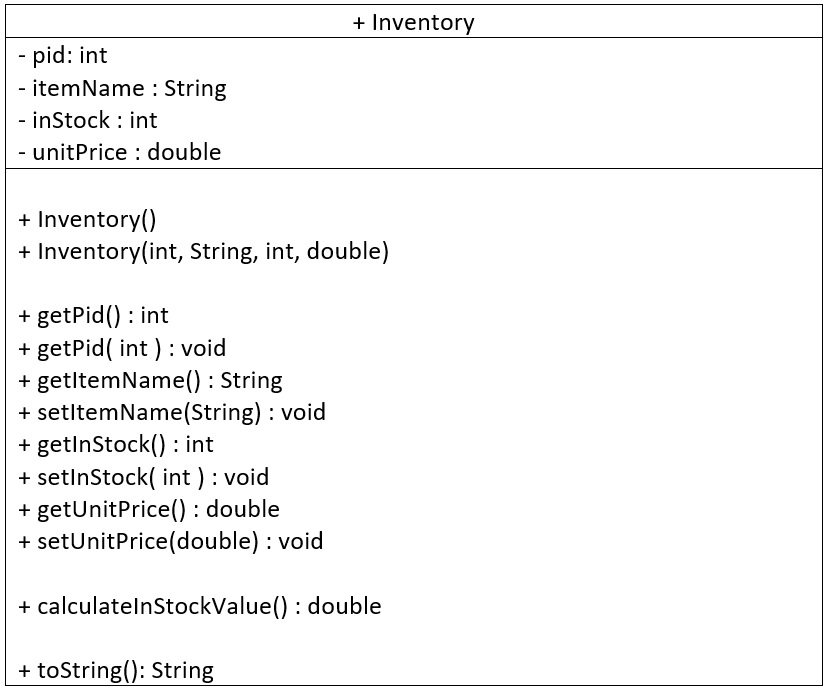
Client program:
populateArray method definition:
printArray method definition:
calculateTotal method definition:
indexOfHighest method definition:
searchItem method definition:
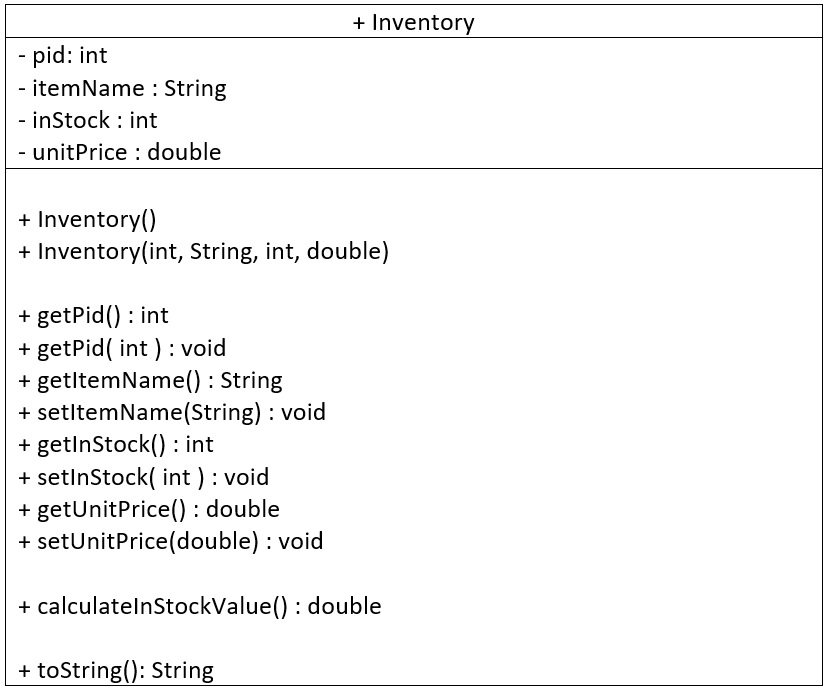
Client program:
Create an array of Inventory
Use JFileChooser object to select the data file
Call populateArray method
Use loop and switch structures to write a menu driven program.
Call printArray method
Call calculateTotal method
Call indexOfHighest method
Call searchItem method
Use JFileChooser object to select the data file
Call populateArray method
Use loop and switch structures to write a menu driven program.
Call printArray method
Call calculateTotal method
Call indexOfHighest method
Call searchItem method
populateArray method definition:
Read the top line of data without storing it
Declare variable i and initialize it to 0
while loop: as long as the data file has data item
Declare variable i and initialize it to 0
while loop: as long as the data file has data item
Read data items from the data file
Create Inventory object and assign it to the array cell i
Increment i
Create Inventory object and assign it to the array cell i
Increment i
printArray method definition:
For i = 0 to last index of array cell
Print array elements at i
calculateTotal method definition:
Declare variable sum and initialize it to 0
For i = 0 to last index of array cell
return sum
For i = 0 to last index of array cell
Add the current element's in-stock value at i to sum
return sum
indexOfHighest method definition:
Declare variable maxIndex and assign it to 0
(Assume the first element has the highest in-stock value)
For i = 1 to last index of array cell
return maxIndex
For i = 1 to last index of array cell
If the in-stock value of current element at i
is greater than the highest element
Update maxIndex to the current index i
Update maxIndex to the current index i
return maxIndex
searchItem method definition:
For i = 0 to last index of array cell
If the loop ended without returning i
Not found and return -1
If the current element at i is equal to the searched key
Found it and return i
Found it and return i
If the loop ended without returning i
Not found and return -1
Hardware Store Data File